Guide
API Design Patterns: Tutorial & Examples
Table of Contents
APIs are the backbone of modern software, enabling communication and data exchange between different systems. They serve as intermediaries, provide critical application functionality, and facilitate interoperability between system components.
Designing effective APIs involves navigating numerous challenges, from ensuring security to managing diverse data formats. API design patterns address these challenges and provide blueprints for efficient, scalable, and maintainable interfaces. This article thoroughly explores API design patterns, including six popular patterns, design recommendations, best practices for API development, and common pitfalls to avoid.
Summary of key API design pattern concepts
The table below summarizes the API design pattern concepts this article will explore in detail.
Concept | Description |
---|---|
Core API design principles | Core principles like consistency, scalability, and flexibility apply across all APIs and are addressed differently by different design patterns. |
API design patterns | Practical strategies like efficient caching, structured pagination, dynamic rate limiting, flexible publish-subscribe systems, and secure access control help to enhance API functionality, manage data flow, and secure API interactions. |
API design tools | Effective API design tools enhance development by providing advanced visualization capabilities, real-time collaboration, automated documentation generation, and version control for API diagrams. |
Core API design principles
A well-structured API architecture ensures robust, scalable, and flexible interactions between different software components. This section delves into the three core principles of API design - consistency, scalability, and flexibility - and explores how design patterns reinforce these principles to create effective APIs.
Consistency
A consistent API provides developers with a predictable and intuitive interface, making it easier to learn and use. Design patterns contribute significantly to this predictability by standardizing the structure of routes, requests, responses, and error handling.
Designing APIs with consistent, standardized endpoint routes helps developers understand and utilize the API more efficiently. For example, a RESTful API typically uses a structured route format to perform CRUD (Create, Read, Update, and Delete) operations. Here’s an example of a TypeScript interface defining a consistent set of CRUD operations for a User
resource:
interface UserRoutes {
createUser: "/api/users"; // POST
getUserById: "/api/users/:id"; // GET
updateUser: "/api/users/:id"; // PUT or PATCH
deleteUser: "/api/users/:id"; // DELETE
}
This pattern of route definition clarifies the available operations and aligns with REST principles by using HTTP methods to indicate the type of those operations.
Likewise, a uniform response structure across an API simplifies handling responses on the client side. Developers can implement more robust and simplified error handling and response parsing by standardizing the contracts around data, status, and errors returned from the API. Below is a TypeScript example of a common API response structure:
interface ApiResponse<T> {
status: "success" | "error";
payload: T;
error?: {
code: string;
message: string;
};
}
In this model, every API response indicates its success or failure through the status
field, carries the primary data in its payload
, and provides an error object with details in case of errors. This standardization ensures that API consumers have a consistent and predictable interface, reducing complexities and potential frustrations in integrating with the API.
In short, implementing these practices - standardized routes and uniform response formats - makes APIs more accessible and reliable, leading to better integration experiences and fewer errors.
Scalability
Scalability means maintaining performance as API usage increases. Scalable APIs utilize strategic implementations like rate limiting and asynchronous architectures, which are crucial for RESTful and non-RESTful interfaces.
Rate limiting
Rate limiting restricts how frequently a user can make requests. Limiting usage rates helps prevent API abuse and ensures that resources are equitably distributed among users, maintaining stable and responsive service during peak traffic periods.
In an Express API, developers can implement rate limiting in middleware with packages like `express-rate-limit` to restrict the number of requests a user can make in a specified period, thereby protecting the API from overuse and potential denial of service attacks.
Here is a TypeScript code snippet demonstrating how to implement this:
import rateLimit from 'express-rate-limit';
import express from 'express';
const app = express();
// Apply rate limiting
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
message: 'Too many requests from this IP, please try again after 15 minutes',
});
// Apply to all API requests
app.use('/api/', apiLimiter);
app.get('/api/example', (req, res) => {
res.send('Request received');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This example sets up a rate limiter that restricts each IP address to 100 requests every 15 minutes, helping to prevent abuse of the API's resources.
A developer platform for system design and architecture documentation
Learn moreAsynchronous architectures
While RESTful APIs are typically associated with synchronous operations, integrating asynchronous patterns can significantly enhance scalability. Asynchronous processes, such as those implemented through webhooks or server-sent events, allow RESTful services to handle tasks like sending real-time notifications without requiring clients to poll the server constantly.
In more dynamic scenarios, leveraging message-based systems for publish/subscribe models, such as WebSockets or event queues, can improve performance. These systems facilitate efficient data handling by asynchronously processing messages, ideal for applications requiring real-time responsiveness and high throughput.
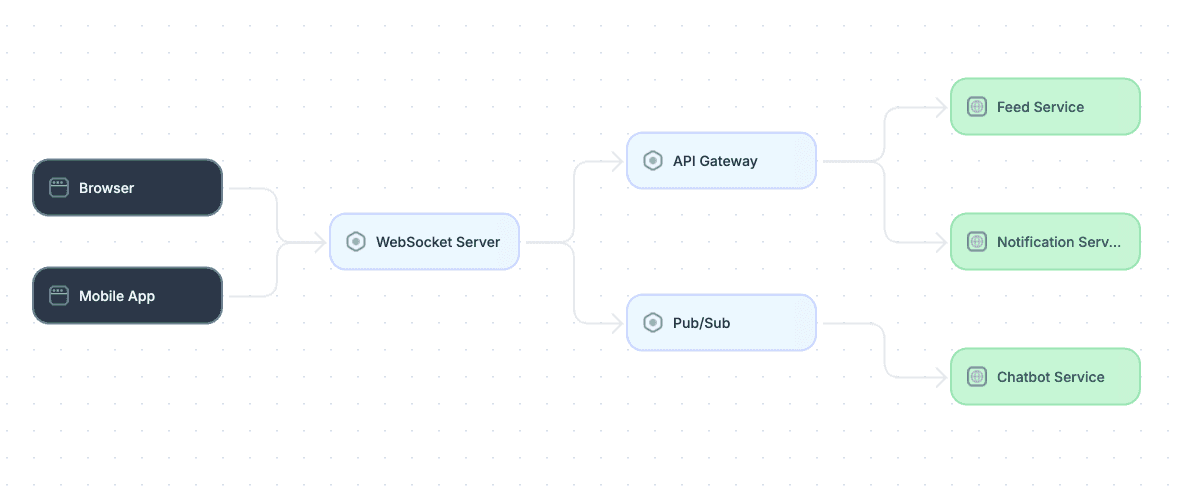
Example social media platform architecture using WebSockets (adapted from source)
Flexibility
Flexibility ensures that APIs can adapt to changes in technology, user needs, and business requirements without disrupting existing client applications. Two effective strategies to achieve this are versioning and using feature flags.
Versioning
Versioning allows APIs to evolve without breaking compatibility with existing clients. In REST APIs, versioning is often achieved by including the version number in the URL path. For instance, the code snippet below provides an example of effective REST API versioning:
import express from 'express';
const app = express();
app.get('/v1/users', (req, res) => {
res.send('Fetching users from version 1');
});
app.get('/v2/users', (req, res) => {
res.send('Fetching users with new feature set from version 2');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
For GraphQL, versioning can be managed by deprecating fields and introducing new ones without impacting existing queries:
type User {
id: ID!
username: String!
email: String! @deprecated(reason: "Use 'contactEmail' instead.")
contactEmail: String
}
Feature flags
Feature flags allow developers to toggle certain features of an API on or off without deploying new code. They can apply to any API paradigm and help test new features in production, control access to specific user segments, or gradually roll out changes.
Here is a TypeScript example demonstrating the implementation of a simple feature flag:
import express from 'express';
const app = express();
const newFeatureActive = true;
app.get('/api/feature', (req, res) => {
if (newFeatureActive) {
res.send('New Feature is enabled');
} else {
res.send('New Feature is disabled');
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Visualize your system architecture for free
Create AccountIn an actual implementation, feature flags are pulled from a database or service (and not hard-coded) to enable changes to API logic without deploying new code. By implementing versioning and feature flags, APIs can evolve dynamically and responsively, ensuring they can meet future demands while maintaining stability for existing users.
API design patterns
API design patterns provide reliable solutions and streamline development, making APIs more robust, scalable, and maintainable. The sections below explain the purpose and implementation of six proven API design patterns.
Efficient caching
- Purpose: To minimize repeated data retrieval operations, improve response times, and reduce load on the API.
- Implementation: Implement HTTP cache headers to manage how responses are stored and revalidated. Utilize the ETag header to make conditional requests to check if the cached data is current, ensuring efficient data delivery and freshness.
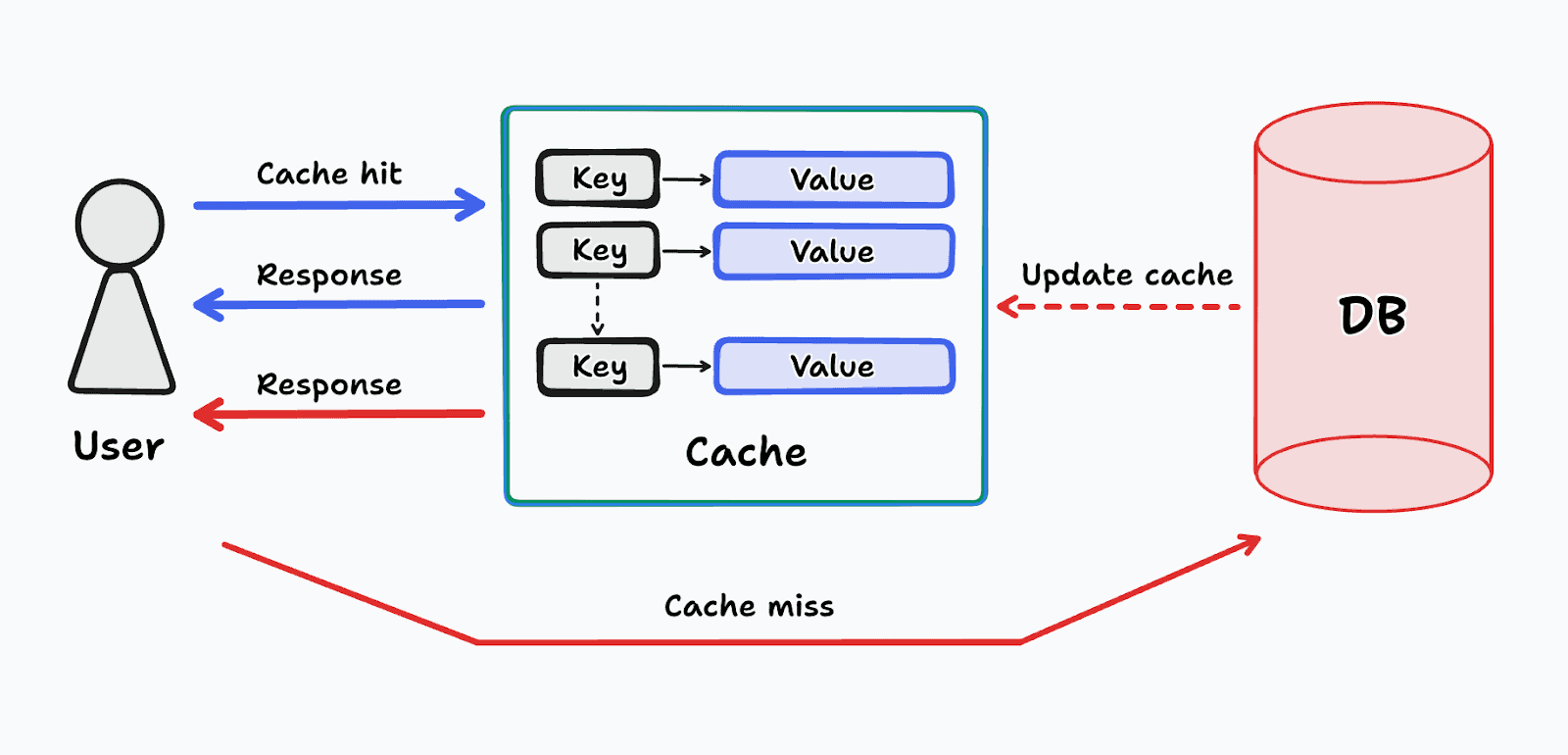
Caching solutions serve frequently accessed data to end users
Structured pagination
- Purpose: To manage large datasets by dividing them into smaller, manageable chunks, ensuring efficient data transfer by reducing the load on the server and the amount of data transferred in each request.
- Implementation: Implement pagination using query parameters like `page` and `pageSize` to allow clients to request specific data portions. Include pagination metadata in API responses to facilitate clients' easy navigation through data pages.
- Code Example: The
ApiResponse
Typescript interface below includes apageInfo
object that provides essential pagination details, such as the current page, total pages, total items, and the number of items per page. This structure can be used across various endpoints in a REST API to provide a consistent and informative experience with paginated data.interface ApiResponse<T> { status: "success" | "error"; payload: T; error?: { code: string; message: string; }; pageInfo?: { currentPage: number; totalPages: number; totalItems: number; itemsPerPage: number; }; }
Dynamic rate limiting
- Purpose: To limit the rate at which users can make requests, preventing API abuse and ensuring service quality and availability.
- Implementation: Choose an appropriate algorithm–such as token bucket or leaky bucket–and implement rate limiting using HTTP headers that communicate the allowed request rate and remaining quota to clients.
Service-level error handling
- Purpose: Protect APIs from cascading failures in distributed systems by temporarily blocking failing operations.
- Implementation: Adopt the Circuit Breaker pattern, which monitors and manages service point failures and circuit interruptions by providing fallback responses or shedding load during downtime.
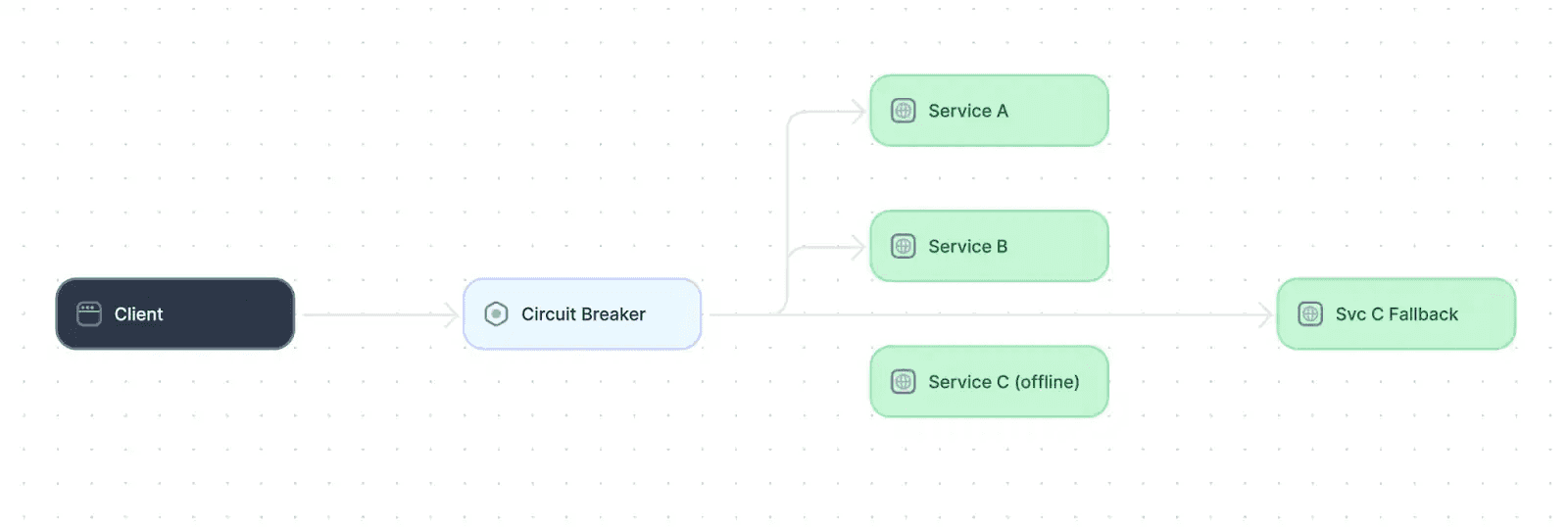
The circuit breaker design pattern common in distributed systems.
Tired of manually updating your system architecture docs?
Sign UpFlexible publish-subscribe systems
- Purpose: To enable event-driven architectures that allow services to publish events that multiple subscribers can react to independently.
- Implementation: Set up topics for message publication and subscription to decouple publishers and subscribers, enhancing scalability and maintainability.
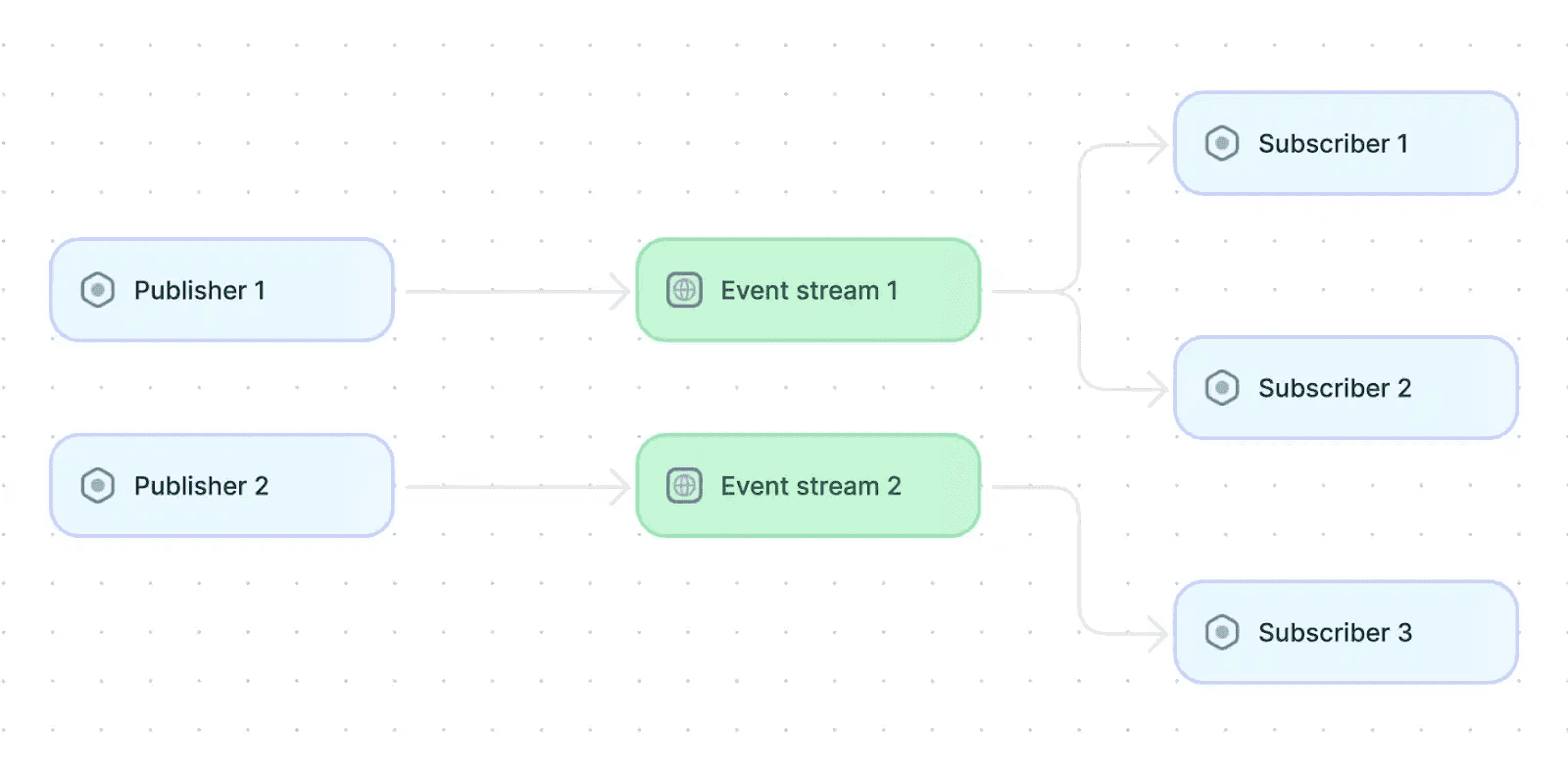
The publisher/subscriber design pattern common in distributed systems.
Secure access control
- Purpose: To verify user identities and enforce permission rules, ensuring only authorized users can access the specified resources.
- Implementation: Integrate robust authentication mechanisms like OAuth and supplement them with thorough authorization checks to manage user and system access levels precisely.
- Code Example: In the Node.js/Express application below, a middleware called
authenticateJWT
checks for a JSON Web Token (JWT) in the Authorization header to verify user access, adding the decoded user to the request object if valid. The setup also includes routes to generate a time-limited JWT upon login and to secure a route that requires a valid JWT, showcasing the basic implementation of JWT for authentication and route protection.import * as express from 'express'; import * as jwt from 'jsonwebtoken'; const app = express(); const secretKey = 'your_secret_key_here'; // Middleware to authenticate JWT tokens const authenticateJWT = ( req: express.Request, res: express.Response, next: express.NextFunction ) => { const authHeader = req.headers.authorization; if (authHeader) { const token = authHeader.split(' ')[1]; jwt.verify(token, secretKey, (err, user) => { if (err) { return res.sendStatus(403); } req.user = user; next(); }); } else { res.sendStatus(401); } }; app.post('/login', (req, res) => { // This should be replaced with real user authentication const { username } = req.body; const user = { name: username }; const accessToken = jwt.sign(user, secretKey, { expiresIn: '1h' }); res.json({ accessToken }); }); app.get('/protected', authenticateJWT, (req, res) => { res.json({ message: 'This is a protected route', user: req.user }); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
Each design pattern above helps to construct effective and reliable APIs. By integrating these patterns strategically, developers can mitigate common API challenges, leading to better application consistency, scalability, flexibility, and performance.
API design tools
Integrating sophisticated design tools into the API design process can accelerate development and amplify the API’s performance, efficiency, and manageability. Effective design tools aid in visualizing complex architectures, facilitating real-time collaboration across teams, managing version control, and automating the creation and management of documentation.
API design tools must handle the complexities of architectural planning, interactive testing, and detailed documentation of APIs. Key technical features expected in such tools include:
- Architecture visualization: A tool should provide advanced visualization features that allow teams to create and manipulate system diagrams easily. These features help maintain an accurate and up-to-date architectural understanding.
- Real-time collaboration: For distributed engineering teams, it is crucial that the tool facilitates synchronous collaboration on design tasks and architecture reviews.
- Version control integration: Tools should integrate smoothly with version control systems to help track changes, manage versions, and ensure everyone in the team can access the latest updates without conflicts.
- Automated and centralized documentation: Automatically generating and maintaining comprehensive documentation from within the tool alleviates the burden of creating these artifacts manually and simplifies the process of keeping all team members aligned and informed.
Tools like Multiplayer include all of these practical features. Multiplayer offers a dynamic and integrated platform to streamline API design and system architecture complexities. In addition, the tool facilitates collaboration among team members, enabling them to share, discuss, and modify system designs in real time.
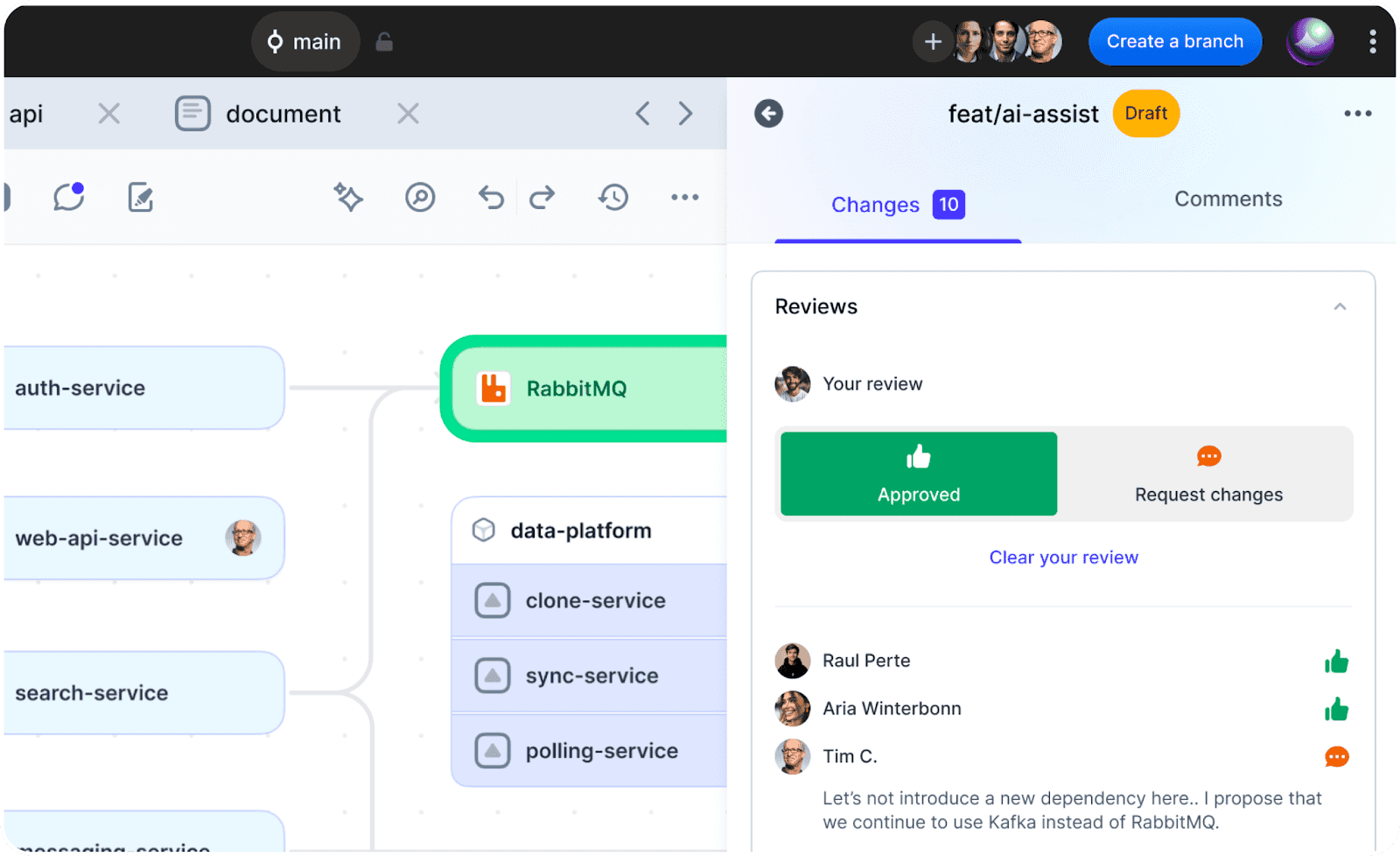
Multiplayer’s UI for collaborative API design reviews
Last thoughts
API design patterns enable teams to use proven solutions to address common API challenges. Teams that adopt these patterns and follow API development best practices can improve the performance and scalability of their APIs. Furthermore, by integrating purpose-built tools like Multiplayer, teams can enhance collaboration throughout the development process, ensuring efficient, secure, and adaptable APIs that serve current system needs and accommodate future growth.